Ultrasonic Sensor (Simulation Mode)
Purpose: The principle of radar based searching can be demonstrated with an ultrasonic sensor. The robot vehicle has the duty to find the direction to a given sound reflecting target and then approach it.
In simulation mode the targets are constructed by a polygon mesh defined from an array of vertex points. A target image may be shown at a given location, but this image is only used for illustration and is not detected as target.
The LegoSim JavaDoc provides more information, e.g. for useTarget():
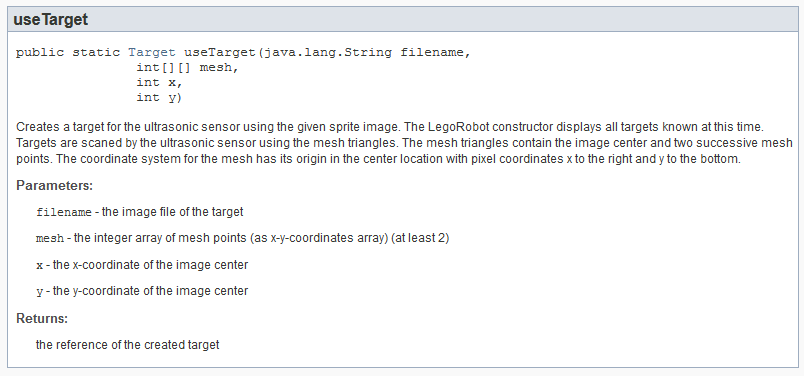
You may nicely animate the radar-like search by displaying a search cone and a proximity circle to the nearest detected target point.
import ch.aplu.robotsim.*;
import java.awt.*;
public class UltrasonicSensorEx1
{
private LegoRobot robot;
private Gear gear;
private UltrasonicSensor us;
public UltrasonicSensorEx1()
{
robot = new LegoRobot();
gear = new Gear();
robot.addPart(gear);
gear.setSpeed(10);
us = new UltrasonicSensor(SensorPort.S1);
robot.addPart(us);
us.setBeamAreaColor(Color.green);
us.setProximityCircleColor(Color.lightGray);
searchTarget();
while (true)
{
if (us.getDistance() < 35)
gear.stop();
}
}
private void searchTarget()
{
while (true)
{
gear.right(50);
int distance = us.getDistance();
if (distance != -1)
{
gear.right(1500);
gear.forward();
return;
}
}
}
public static void main(String[] args)
{
new UltrasonicSensorEx1();
}
// ------------------ Environment --------------------------
static
{
Point[] mesh =
{
new Point(50, 0), new Point(25, 42), new Point(-25, 42),
new Point(-50, 0), new Point(-25, -42), new Point(25, -42)
};
RobotContext.useTarget("sprites/target_red.gif", mesh, 380, 380);
}
}
Execute the program locally using WebStart.
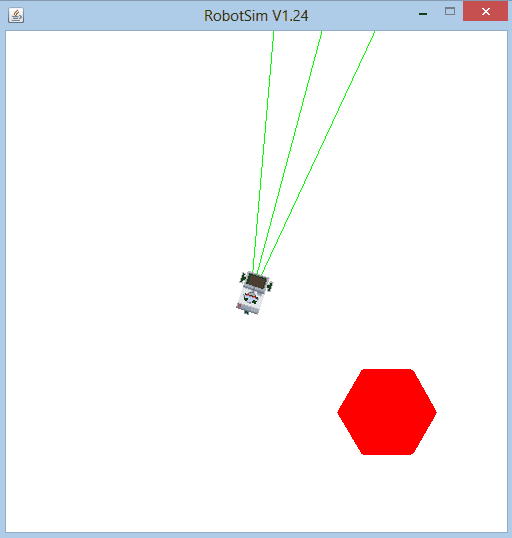
Discussion: You can easily port this program to the autonomous or direct mode. The environment then becomes a real sound reflecting target like a hexagonal box standing on the floor.
|