|
In the following tutorial we introduce to the fundamental ideas of the JCardGame framework. The paradigm of "Learning by Examples" is applied where each little example is fully functional and has a special didactical intension. The examples are not yet real games to play with, but serves as demonstration of a specific feature of JCardGame. All sources and sprite images are included in the JCardGame distribution. |
Ex10: Searching for Pairs, Trips and Quads
In many card game a hand with two (pairs), three (trips) or even four cards (quads) of the same rank is of special merit. You search a given hand for these special combination using extractPairs(), extractTrips() and extractQuads(). It is important to mention that if a card is part of a quad, it is not considered to be part of a trip or a pair. If a card is part of a trip, it is not considered to be part of a pair. All three methods returns a array of hands that contains the card references (and not card clones).
In the following example a master hand with 25 cards is created and displayed. Clicking into the card game window extracts pairs, trips and quads from the master hand and displays them in the lower part of the window. The next mouse click creates another master hand of 25 cards. It is important to cleanup all the hands before getting the new master hand. To halt the program execution and wait for the mouse click, Monitor.putSleep()/wakeUp() is used.
import ch.aplu.jcardgame.*;
import ch.aplu.jgamegrid.*;
import ch.aplu.util.Monitor;
public class Ex10 extends CardGame implements GGMouseListener
{
public enum Suit
{
SPADES, HEARTS, DIAMONDS, CLUBS
}
public enum Rank
{
ACE, KING, QUEEN, JACK, TEN, NINE, EIGHT, SEVEN, SIX
}
private Deck deck = new Deck(Suit.values(), Rank.values(), "cover");
public Ex10()
{
super(900, 615, 30);
addMouseListener(this, GGMouse.lPress);
while (true)
{
Hand hand = deck.dealingOut(1, 25)[0];
RowLayout rowLayout = new RowLayout(new Location(450, 80), 890);
hand.setView(this, rowLayout);
hand.sort(Hand.SortType.RANKPRIORITY, true);
hand.draw();
setStatusText("Click to get pairs, trips and quads");
Monitor.putSleep();
Hand[] pairs = hand.extractPairs();
for (int i = 0; i < pairs.length; i++)
{
pairs[i].
setView(this, new RowLayout(new Location(70 + 150 * i, 230), 120));
pairs[i].draw();
}
Hand[] trips = hand.extractTrips();
for (int i = 0; i < trips.length; i++)
{
trips[i].
setView(this, new RowLayout(new Location(70 + 150 * i, 380), 120));
trips[i].draw();
}
Hand[] quads = hand.extractQuads();
for (int i = 0; i < quads.length; i++)
{
quads[i].
setView(this, new RowLayout(new Location(100 + 200 * i, 530), 150));
quads[i].draw();
}
setStatusText("Click to generate the next card set.");
Monitor.putSleep();
// Cleanup all hands
hand.removeAll(false);
for (Hand h : pairs)
h.removeAll(false);
for (Hand h : trips)
h.removeAll(false);
for (Hand h : quads)
h.removeAll(false);
}
}
public boolean mouseEvent(GGMouse mouse)
{
Monitor.wakeUp();
return true;
}
public static void main(String[] args)
{
new Ex10();
}
} |
Execute the program locally using WebStart.
 |
Download Android app for installation on a smartphone or emulator.
Download sources (CardEx10.zip).
Create QR code to download Android app to your smartphone.
Install/Start app on a USB connected smartphone or a running emulator.
(This is a WebStart signed by the University of Berne, Switzerland. It installs some helper files in <userhome>.jdroidtools.If you did not install the Android SDK, you may install a slim version of the Android-Emulator in <userhome>.jdroidemul using this link, To start the emulator, execute ExecEmul.jar found in <userhome>.jdroidemul) |
|
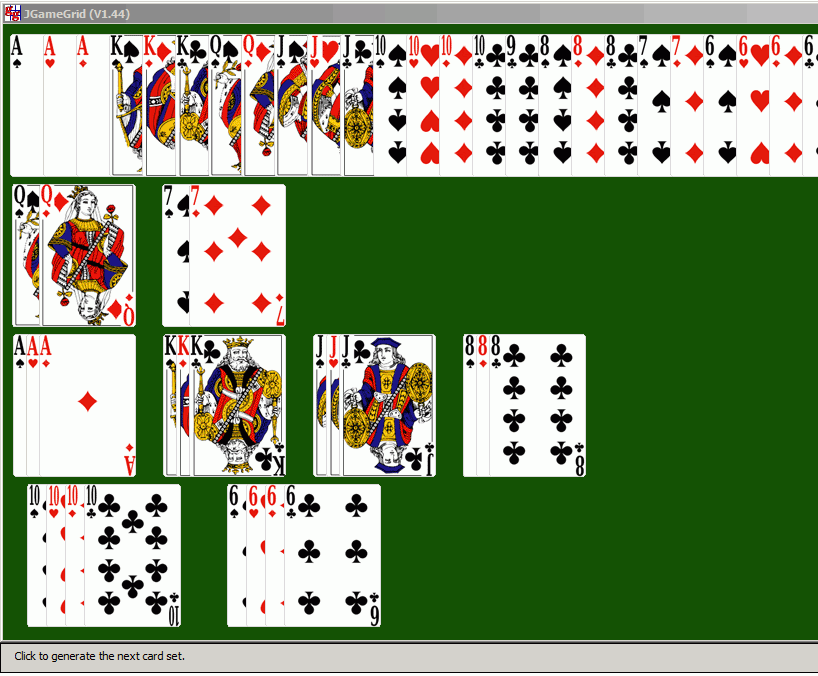
Ex11: Searching for Sequences
Searching for cards with succeeding rank (sometimes called a straight) is required for many card games. Normally the cards must have the same suit to be counted as a sequence, but there are games where the suits can be mixed. The Hand class provides methods extractSequences() that performs a search for sequences of the same or mixed suits. Because a sequence may be considered as a hand, extractSequences() returns an array of hands. The current hand remains unchanged and the cards in the returned hands have their suit and rank copied, but no card actor is associated. You should be careful when using these hands in order to avoid card duplication. In the following example a deck with 25 random cards is searched for sequences of length 3, 4 and 5. The original deck and the sequences are shown in the card game window. Whenever you click into the window, a next deck is created and analyzed.
import ch.aplu.jcardgame.*;
import ch.aplu.jgamegrid.*;
import ch.aplu.util.Monitor;
public class Ex11 extends CardGame implements GGMouseListener
{
public enum Suit
{
SPADES, HEARTS, DIAMONDS, CLUBS
}
public enum Rank
{
ACE, KING, QUEEN, JACK, TEN, NINE, EIGHT, SEVEN, SIX, FIVE, FOUR, THREE, TWO
}
private Deck deck = new Deck(Suit.values(), Rank.values(), "cover");
public Ex11()
{
super(900, 615, 30);
addMouseListener(this, GGMouse.lPress);
while (true)
{
Hand hand = deck.dealingOut(1, 25)[0];
hand.setView(this, new RowLayout(new Location(450, 80), 890));
hand.sort(Hand.SortType.RANKPRIORITY, true);
Hand[] sequence3 = hand.extractSequences(Suit.HEARTS, 3);
for (int i = 0; i < sequence3.length; i++)
{
sequence3[i].
setView(this, new RowLayout(new Location(70 + 150 * i, 230), 120));
sequence3[i].draw();
}
Hand[] sequence4 = hand.extractSequences(Suit.HEARTS, 4);
for (int i = 0; i < sequence4.length; i++)
{
sequence4[i].
setView(this, new RowLayout(new Location(70 + 150 * i, 380), 120));
sequence4[i].draw();
}
Hand[] sequence5 = hand.extractSequences(Suit.HEARTS, 5);
for (int i = 0; i < sequence5.length; i++)
{
sequence5[i].
setView(this, new RowLayout(new Location(100 + 200 * i, 530), 150));
sequence5[i].draw();
}
setStatusText("Click to generate the next card set.");
Monitor.putSleep();
// Cleanup all hands
hand.removeAll(false);
for (Hand h : sequence3)
h.removeAll(false);
for (Hand h : sequence4)
h.removeAll(false);
for (Hand h : sequence5)
h.removeAll(false);
}
}
public boolean mouseEvent(GGMouse mouse)
{
Monitor.wakeUp();
return true;
}
public static void main(String[] args)
{
new Ex11();
}
} |
Execute the program locally using WebStart.
 |
Download Android app for installation on a smartphone or emulator.
Download sources (CardEx11.zip).
Create QR code to download Android app to your smartphone.
Install/Start app on a USB connected smartphone or a running emulator.
(This is a WebStart signed by the University of Berne, Switzerland. It installs some helper files in <userhome>.jdroidtools.If you did not install the Android SDK, you may install a slim version of the Android-Emulator in <userhome>.jdroidemul using this link, To start the emulator, execute ExecEmul.jar found in <userhome>.jdroidemul) |
|
Searching for mixed sequences is exactly the same from the user point of view. You just take the overloaded version of extractSequences() with no suit parameter. The internal algorithm is more complicated because all of the possibilities for mixed sequences must be checked.
Execute the program locally using WebStart. Click into the window to generate another deck.
 |
Download Android app for installation on a smartphone or emulator.
Download sources (CardEx11a.zip).
Create QR code to download Android app to your smartphone.
Install/Start app on a USB connected smartphone or a running emulator.
(This is a WebStart signed by the University of Berne, Switzerland. It installs some helper files in <userhome>.jdroidtools.If you did not install the Android SDK, you may install a slim version of the Android-Emulator in <userhome>.jdroidemul using this link, To start the emulator, execute ExecEmul.jar found in <userhome>.jdroidemul) |
|
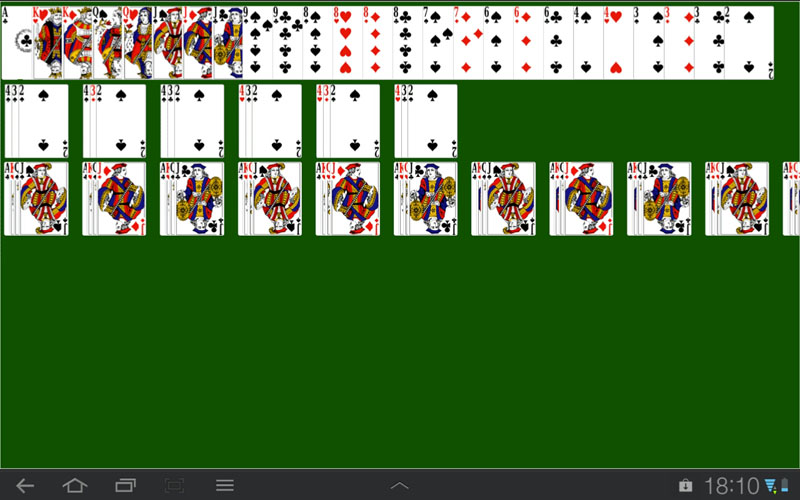
| |